Key Takeaway:
Explore 5 Python methods (Python Average) for list average computation, optimizing efficiency and precision, including built-in functions and NumPy.
Introduction:
Uncover efficient Python approaches to computing list averages, leveraging diverse methods for accuracy and performance.
5 Ways to Find the Average of a List in Python
To find the average of a list in Python, you can employ various techniques:
- Python's mean() function: Python 3 includes a statistics module with a mean() function, which calculates the average of input values or a dataset.
Syntax: mean(data-set/input-values)
Example:
from statistics import mean
inp_lst = [12, 45, 78, 36, 45, 237.11, -1, 88]
list_avg = mean(inp_lst)
print("Average value of the list:\n")
print(list_avg)
print("Average value of the list with precision up to 3 decimal places:\n")
print(round(list_avg,3))
- Using Python's sum() function: Python's sum() function sums all data items in a list, and by dividing this sum by the count of items, you can obtain the average.
Example:
from statistics import mean
inp_lst = [12, 45, 78, 36, 45, 237.11, -1, 88]
sum_lst = sum(inp_lst)
lst_avg = sum_lst/len(inp_lst)
print("Average value of the list:\n")
print(lst_avg)
print("Average value of the list with precision up to 3 decimal places:\n")
print(round(lst_avg,3))
- Using Python's reduce() and lambda: Python's reduce() function, along with lambda, can be used to iteratively apply a function to the elements of a list.
Example:
from functools import reduce
inp_lst = [12, 45, 78, 36, 45, 237.11, -1, 88]
lst_len= len(inp_lst)
lst_avg = reduce(lambda x, y: x + y, inp_lst) /lst_len
print("Average value of the list:\n")
print(lst_avg)
print("Average value of the list with precision up to 3 decimal places:\n")
print(round(lst_avg,3))
- Using Python's operator. add() function: Python's operator module includes the add() function, which, combined with reduce(), can calculate the sum of all list elements.
Example:
from functools import reduce
import operator
inp_lst = [12, 45, 78, 36, 45, 237.11, -1, 88]
lst_len = len(inp_lst)
lst_avg = reduce(operator.add, inp_lst) /lst_len
print("Average value of the list:\n")
print(lst_avg)
print("Average value of the list with precision up to 3 decimal places:\n")
print(round(lst_avg,3))
- NumPy's average() method: NumPy provides an average() method to compute the mean of a list efficiently.
Example:
import numpy
inp_lst = [12, 45, 78, 36, 45, 237.11, -1, 88]
lst_avg = numpy.average(inp_lst)
print("Average value of the list:\n")
print(lst_avg)
print("Average value of the list with precision up to 3 decimal places:\n")
print(round(lst_avg,3))
Introduction to Finding Average in Python
Welcome to the world of Python Average, where numbers come alive and calculations become a breeze! If you've ever wondered how to find the average of a list in Python, then you're in for a treat. In this blog post, we will dive into the art of calculating averages using Python's powerful coding capabilities.
Whether you're a seasoned programmer or just starting your journey in the world of coding, finding the average is an essential skill that can be applied to various real-life scenarios. From analyzing data sets to measuring performance metrics, understanding how to calculate averages is crucial for making informed decisions.
So grab your favorite beverage and get ready to embark on this exciting adventure as we explore different methods for finding averages in Python. By the end of this article, you'll have all the tools necessary to conquer any numerical challenge that comes your way!
But before we delve deeper into our quest for averaging greatness, let's take a moment to understand some basic concepts about lists and data types in Python. So buckle up and let's get started!
Understanding Lists and Data Types in Python
When working with data in Python, it's important to understand the concept of lists and data types. In Python, a list is an ordered collection of elements that can contain different types of data such as numbers, strings, or even other lists. This flexibility makes lists a powerful tool for organizing and manipulating data.
Data types in Python refer to the classification or categorization of variables based on their nature and characteristics. Some common data types include integers (whole numbers), floats (decimal numbers), strings (text), booleans (True or False values), and more.
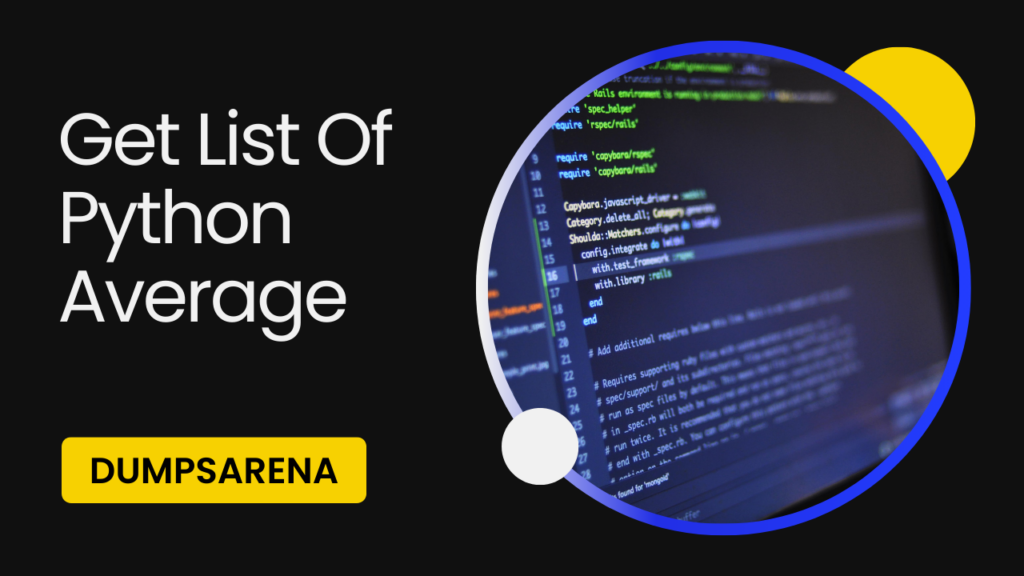
Lists are denoted by square brackets [] and each element is separated by a comma. For example:
my_list = [1, 2, 3, "hello", True]
One key aspect to remember about lists is that they are mutable, meaning that you can modify their contents after they have been created. This allows for dynamic manipulation of data within a list.
To access individual elements within a list, you can use indexing starting from zero. For example:
my_list = [10, 20, 30]
print(my_list[0]) # Output: 10
Python also provides various built-in functions that help manipulate lists easily. These functions include len(), which returns the length of a list; append(), which adds an element at the end of a list; remove(), which deletes an element from a list; sort(), which arranges elements in ascending order; and many more!
Understanding how lists work along with knowledge about the different data types will pave your way towards effectively calculating averages using Python! So let's dive into some methods for finding the average next!
Methods for Calculating Averages in Python
Calculating the average of a list is a common task in data analysis and programming. Thankfully, Python provides several methods to help us simplify this process. Let's explore some of these methods and see how they can be used.
One straightforward approach is to use a for loop to iterate through the list elements and calculate the sum. We can then divide the sum by the length of the list to obtain the average. This method allows us to handle lists of any size, making it quite versatile.
Another helpful tool in Python is the statistics module, which offers functions specifically designed for statistical calculations. By using functions like mean() or mean (), we can easily find averages without having to write complex code from scratch.
Additionally, if you're working with large datasets or need more precise calculations, there are other options available such as numpy and pandas libraries. These libraries provide powerful tools for data manipulation and analysis, including efficient ways to calculate averages.
Remember that when dealing with numeric values stored as strings in a list, you may need to convert them into integers or floats before performing calculations. Otherwise, you might encounter unexpected errors or incorrect results.
By familiarizing yourself with these different methods for calculating averages in Python, you'll have more flexibility when working on your projects and analyzing data sets efficiently.
Continue reading our blog post series on Python Average where we delve deeper into each method discussed here and highlight their advantages depending on specific use cases. Stay tuned!
Using a For Loop to Find Python Average
To calculate the average of a list in Python, one approach is to use a for loop. This method allows you to iterate through each element in the list and keep track of their total sum.
First, you need to initialize two variables: one for storing the sum of all elements and another for keeping count of how many elements are in the list. You can set both variables to zero before starting the loop.
Next, use a for loop to iterate over each element in the list. Inside the loop, add each element to the sum variable and increment the count variable by one.
Python Average Once the loop completes, divide the sum by count to get the average value. Remember that dividing integers may result in an integer quotient, so it's important to convert either the numerator or denominator (or both) into floats if decimal precision is desired.
You can print or return this average value as per your requirement.
Using a for loop provides flexibility when dealing with lists of different sizes and allows you complete control over calculating averages without relying on external libraries or modules.
Utilizing the Statistics Module for More Advanced Calculations
Python Average provides a powerful module called "statistics" that can be used to perform more advanced calculations, including finding the average of a list. This module offers a range of statistical functions that can save you time and effort when working with data.
To get started, you'll need to import the statistics module into your Python script or interactive session. Simply use the following line of code: import statistics
.
Once imported, you have access to various functions such as mean(), median(), mode(), and stdev(). These functions allow you to calculate different measures of central tendency and dispersion in your data.
For example, if you have a list of numbers stored in a variable called "data", you can easily find the average using the mean() function like this: average = statistics.mean(data)
.
The advantage of using these built-in functions is that they handle all the calculations for you, saving you from implementing complex algorithms manually. Plus, they are optimized for performance and accuracy.
In addition to calculating averages, the statistics module also enables other useful operations like finding medians (the middle value), modes (the most frequently occurring values), and standard deviations (a measure of how spread out your data is).
By taking advantage of these features, you can gain deeper insights into your datasets without having to reinvent the wheel. The flexibility offered by Python's statistics module allows for efficient analysis and exploration of your data.
Remember that before applying any statistical calculations to your dataset, it's important to ensure its integrity by handling missing values or outliers appropriately. Data cleaning and preprocessing are crucial steps in obtaining accurate results.
In conclusion,
The statistics module in Python provides convenient methods for performing advanced calculations on lists or datasets. By importing this module into your code, you gain access to functions such as mean(), median(), mode(), and stdev() which enable efficient calculation of various statistical measures. Leveraging the statistics module allows you to save time and effort while ensuring accurate results. However
Common Errors and Troubleshooting Tips
Python Average When working with Python, it's not uncommon to encounter some errors or face challenges while trying to find the average of a list. But fear not! We've got you covered with some handy troubleshooting tips to help you overcome these obstacles.
One common error that beginners often encounter is forgetting to convert their input values to integers before performing calculations. Remember, Python treats input as strings by default, so if you're working with numerical data, make sure to convert it using the int() function.
Another issue that can arise is dealing with empty lists. If your list doesn't have any elements, attempting to calculate the average will result in an error. To avoid this, you can add a simple check at the beginning of your code using an if statement. If the list is empty, handle it accordingly (e.g., display a message or set a default value).
Furthermore, keep an eye out for typos or syntax errors in your code. One small mistake like misspelling "sum" as "suM" can lead to unexpected results or even cause your program to crash entirely!

Python Average Be mindful of dividing by zero when calculating averages. This may happen if all the values in your list are zeros or if there are no valid numbers present at all. You can address this issue by checking for zero denominators before performing division operations.
By being aware of these common errors and following these troubleshooting tips, you'll be well-equipped to tackle any issues that come your way while finding the average of a list in Python!
Remember - practice makes perfect! So don't get discouraged if you stumble upon these challenges initially; with time and experience comes mastery over such issues!
Conclusion and Additional Resources
Python Average In this article, we explored different methods for finding the average of a list in Python. We started by understanding lists and data types in Python, which laid the foundation for our calculations. Then, we discussed two main approaches: using a for loop to manually calculate the average and utilizing the statistics module for more advanced calculations.
Using a for loop is a straightforward method that allows you to iterate through each element of the list and sum them up. By dividing the total sum by the number of elements, you can find the average. This approach is useful when you want to have control over each step of the calculation process.
On the other hand, if you're looking for more advanced statistical calculations or dealing with large datasets, using Python's statistics module can be highly advantageous. It provides various functions such as mean(), median(), mode(), and stdev() that simplify complex statistical operations.
Python Average While working with averages in Python, it's important to watch out for common errors such as division by zero or handling non-numeric values in your list. Troubleshooting these issues can save you time and ensure accurate results.
To further enhance your knowledge of working with averages in Python, here are some additional resources:
- Official documentation on lists: [https://shorturl.at/bRUV7]
- Statistics module documentation: [https://shorturl.at/bRUV7]
- Stack Overflow - an online community where programmers share their experiences and ask questions related to coding: [https://shorturl.at/bRUV7]
Remember that practice makes perfect when it comes to programming skills like calculating averages in Python. Keep experimenting with different scenarios and datasets to deepen your understanding.
So go ahead and put your newfound knowledge into action! Happy coding!
Comments (0)