Top 100 Java Coding Interview Questions Easy To Crack Java Coding
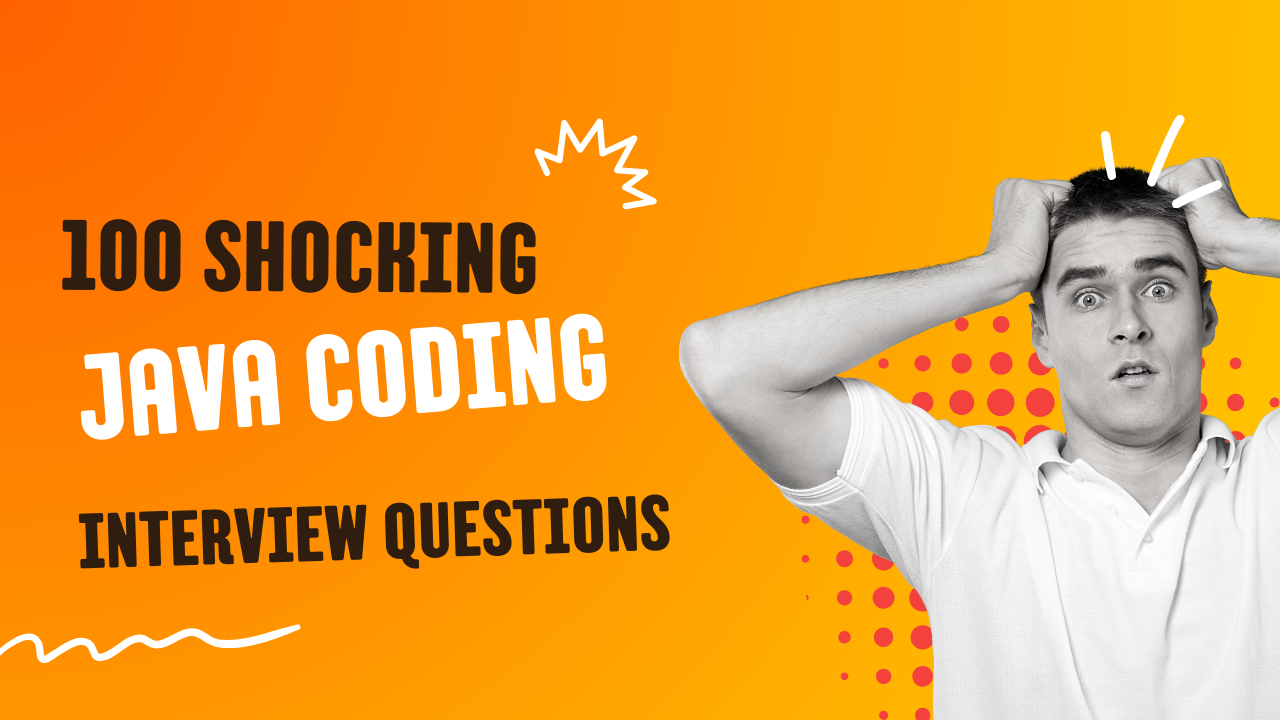
Key Takeaway:
Java Coding Interview Questions Explore essential Java interview programs with solutions, covering string manipulation, array operations, mathematical challenges, and collection-related tasks.
Introduction:
Dive into a comprehensive collection of Java interview programs, unraveling solutions for common coding challenges, array manipulations, and diverse mathematical problems.
Certainly! Below is the trimmed and refined version of your list:
1) Reverse a String in Java
Write a Java program to reverse a given string using a recursive method.
2) Create a Pyramid of Numbers in Java
Write a Java program to create a pyramid of numbers or symbols in pyramid patterns.
3) Remove All White Spaces from a String in Java
Write a Java program to remove all white spaces from a given string.
4) Find Duplicate Characters in a String in Java
Write a Java program to find duplicate characters and their count in a given string.
5) Check Equality of Two Arrays in Java
Write a Java program to check the equality of two arrays using different methods.
6) Anagram Program in Java
Write a Java program to check whether two given strings are anagrams or not.
7) Armstrong Number Program in Java
Write a Java program to check whether a given number is an Armstrong number or not.
8) Find Duplicate Elements in an Array
Write a Java program to find duplicate elements in a given array using five different methods.
9) Find Sum of All Digits of a Number in Java
Write a Java program to find the sum of all digits of a given number.
10) Find Second Largest Number in an Integer Array
Write a Java program to find the second largest number in an array of numbers.
11) Perform Matrix Operations in Java
Write a Java program to perform matrix operations such as addition, subtraction, multiplication, and transpose.
12) Count Occurrences of Each Character in a String
Write a Java program to count the number of occurrences of each character in a given string.
13) Find Largest Number Less Than a Given Number and Without a Given Digit
Write a Java program to find the largest number less than a given number that does not contain a specific digit.
14) Find All Pairs of Elements in an Array Whose Sum is Equal to a Given Number
Write a Java program to find all pairs of elements in an array whose sum is equal to a given number.
15) Find Continuous Subarray Whose Sum is Equal to a Given Number
Write a Java program to find a continuous subarray in a given array whose elements add up to a specified number.
16) Remove Duplicate Elements from ArrayList in Java
Write a Java program to remove duplicate elements from an ArrayList.
17) Check Whether a Given Number is Binary or Not
Write a Java program to check whether a given number is binary or not.
18) Check Whether One String is a Rotation of Another in Java
Write a Java program to check whether one string is a rotation of another.
19) Find the Intersection of Two Arrays in Java
Write a Java program to find the intersection of two arrays or common elements between them.
20) Check Whether User Input is a Number or Not in Java
Write a Java program to check whether user input is a number or not.
50) Stop a Thread in Java
Write a Java program to stop a thread.
51) Remove Duplicate Elements from an Array
Write a Java program to remove duplicate elements from a given array.
52) Append Text to a File in Java
Write a Java program to append text to a file.
53) Synchronize ArrayList, HashSet, and HashMap in Java
Write a Java program to synchronize ArrayList, HashSet, and HashMap.
54) Find Number of Characters, Words, and Lines in a Text File
Write a Java program to find the number of characters, words, and lines in a text file.
55) Convert HashMap to ArrayList in Java
Write a Java program to convert HashMap to ArrayList.
56) Sort an ArrayList in Java
Write a Java program to sort an ArrayList.
57) All Permutations of a String
Write a Java program to find all permutations of a string recursively.
58) Check if a Number Belongs to Fibonacci Series or Not
Write a Java program to check whether a given number belongs to the Fibonacci series.
59) 15 Java HashMap Programming Examples
60) Print Floyd’s Triangle in Java
Write a Java program to print Floyd’s Triangle.
61) Find First Repeated and Non-Repeated Character in a String
Given a string, find the first repeated and non-repeated character.
62) Spiral Matrix Program
Write a Java program to create a spiral matrix of numbers in both clockwise and anti-clockwise directions.
63) String to Integer and Integer to String Conversion Program
64) Array Element Removal Programs
Write Java programs for various array element removal scenarios using the Apache Commons Lang library.
Feel free to continue the list or ask if you have any specific questions!
Introduction To Java Coding Interview Questions
Welcome to the world of Java coding interviews! If you’re gearing up for a job interview in the tech industry, chances are you’ll encounter questions that put your Java skills to the test. To help you prepare and boost your confidence, we’ve compiled the ultimate list of the top 100 Java coding interview questions.
But why is preparation so crucial? Well, just like any other field, practice makes perfect in programming. The more familiar you are with common Java concepts, data structures and algorithms, object-oriented programming principles, as well as popular libraries and frameworks – the better equipped you’ll be to tackle those challenging interview questions.
In this blog post, we’ll cover everything from basic Java concepts to advanced topics like debugging and troubleshooting. Whether you’re a beginner or an experienced developer looking to brush up on your skills before an interview, our comprehensive guide will provide valuable insights and tips to help you pass with flying colors.
So grab your favorite beverage (coffee anyone?), sit back, and let’s dive into our curated list of top 100 Java coding interview questions. By mastering these concepts and practicing regularly with our prep material, you’ll soon be ready to showcase your expertise in any Java coding interview that comes your way!
Why Preparation is Key for Java Coding Interviews?
Preparing for a Java coding interview is crucial if you want to stand out from the competition and land that dream job. It’s not enough to simply have a basic knowledge of Java; you need to be well-prepared and ready to tackle any question that comes your way.
Preparing beforehand allows you to familiarize yourself with the most commonly asked questions in Java coding interviews. By studying these questions and practicing their solutions, you’ll gain confidence in your abilities and be better equipped to handle similar problems during the actual interview.
Preparation helps sharpen your problem-solving skills. Many Java coding interview questions are designed to test your ability to think critically and come up with efficient solutions. By practicing these types of questions, you’ll become more adept at breaking down complex problems into manageable steps and finding optimal solutions.
Additionally, preparation allows you to brush up on important concepts such as object-oriented programming (OOP), data structures, algorithms, and key libraries/frameworks used in Java development. A solid understanding of these topics will greatly enhance your performance during the interview.
Furthermore, being prepared allows you to showcase your expertise confidently. Interviewers often look for candidates who can demonstrate deep knowledge of Java concepts and apply them effectively. With thorough preparation, you can highlight your skills by providing clear explanations and well-structured code examples.
Last but perhaps most importantly – preparation reduces stress! Walking into an interview without adequate preparation can significantly increase nervousness and anxiety levels. However, when armed with knowledge gained through practice sessions or mock interviews, you’ll feel much calmer knowing that you’ve put in the effort required for success.
In conclusion: Preparation is key when it comes to excelling in a Java coding interview. Through diligent study, practice exercises, reviewing key concepts, and gaining confidence along the way, you’ll position yourself as a strong candidate capable of tackling any challenge thrown at you. So, don’t underestimate the importance of preparation – it could be the difference
The Top 100 Java Coding Interview Questions and Answers
When it comes to preparing for a Java coding interview, having a solid understanding of the most commonly asked questions is essential. Whether you are a beginner or an experienced developer, being well-prepared can make all the difference in landing your dream job.
In this section, we will explore some of the top 100 Java coding interview questions and provide brief answers to help you get started. Please note that these questions cover various topics ranging from basic concepts to advanced data structures and algorithms, so be sure to brush up on your knowledge before diving in.
- What is Java?
Java is a high-level programming language widely used for developing applications across different platforms. - Explain the main features of Java.
Java offers features like platform independence, object-oriented programming support, automatic memory management, and robust exception handling. - What are the differences between abstract classes and interfaces?
An abstract class can have both declared methods with implementation and abstract methods, while an interface only allows method declarations without any implementations. - How does multithreading work in Java?
Multithreading allows multiple threads to execute concurrently within a single program by dividing tasks into smaller units called threads. These threads then run independently but share resources like memory space. - Can you explain what garbage collection is in Java?
Garbage collection is an automatic process where unused objects are identified and removed from memory by the JVM (Java Virtual Machine) to free up space for new objects. - What are some popular libraries/frameworks used in Java development?
Some popular libraries/frameworks include Spring Framework, Hibernate ORM (Object-Relational Mapping), Apache Struts framework, JUnit for unit testing, Log4j for logging purposes,
A. Basic Java Concepts
Java is one of the most popular programming languages used worldwide, and having a strong understanding of its basic concepts is essential for acing any Java coding interview. Here are some key topics you should familiarize yourself with:
- Variables and Data Types:
In Java, variables are used to store data, and each variable has a specific data type such as int, double, or String. Understanding how to declare and use variables correctly is crucial. - Control Flow Statements:
These statements allow you to control the flow of execution in your program by using conditional statements like if-else and loops like for and while. - Object-oriented Programming (OOP):
OOP is a fundamental concept in Java where you create objects that have properties (variables) and behaviors (methods). It includes concepts like classes, inheritance, polymorphism, and encapsulation. - Exception Handling:
Exceptions occur when something unexpected happens during program execution. Knowing how to handle exceptions gracefully can prevent your program from crashing. - Input/Output Operations:
Being able to read input from the user or write output to a file is an important skill in Java coding. - Memory Management:
Understanding how memory allocation works in Java helps optimize your code’s efficiency by managing resources effectively. - Strings:
Manipulating strings efficiently using methods like concatenation or substring operations can be important in many coding scenarios.
Remember that these are just some basic concepts within the vast world of Java programming!
B. Object-Oriented Programming
Object-oriented programming (OOP) is a fundamental concept in Java and plays a crucial role in coding interviews. It revolves around the idea of creating objects that encapsulate data and behavior, promoting code reusability and flexibility. Here are some key points to keep in mind:
- Inheritance:
Inheritance allows classes to inherit properties and methods from other classes, providing a way to create hierarchical relationships. It promotes code reuse by enabling subclasses to inherit common attributes and behaviors from their parent class. - Polymorphism:
Polymorphism allows objects of different classes to be treated as objects of a common superclass, providing flexibility in method implementation at runtime. This feature helps achieve loose coupling and enhances extensibility. - Abstraction:
Abstraction involves simplifying complex systems by breaking them down into manageable components or abstract classes/interfaces with defined behaviors but incomplete implementations. It enables code modularity, making it easier to maintain and understand. - Encapsulation:
Encapsulation refers to bundling data (attributes) together with methods that operate on that data within an object’s boundary using access modifiers like public, private, protected, etc., ensuring data security while allowing controlled access. - Interfaces vs Abstract Classes:
Both interfaces and abstract classes provide mechanisms for abstraction; however, there are differences between them regarding usage scenarios, multiple inheritance support, default method implementations (in interfaces), etc.
Understanding these concepts is vital for tackling OOP-related questions during coding interviews effectively!
C. Data Structures and Algorithms
Data structures and algorithms are fundamental concepts in computer science, and mastering them is essential for any Java developer hoping to succeed in coding interviews. Here, we will dive into some of the most commonly asked questions related to data structures and algorithms.
One popular topic is linked lists. Interviewers often ask about implementing a linked list or performing operations on it, such as inserting or deleting nodes. It’s crucial to understand how linked lists work and be able to write clean code for these tasks.
Another important area is sorting algorithms. You should be familiar with different sorting techniques like bubble sort, selection sort, insertion sort, merge sort, and quicksort. Understanding their time complexity and when to use each one is vital.
Binary trees also frequently appear in coding interviews. You may be asked about traversing a tree using depth-first search (DFS) or breadth-first search (BFS), finding the height of a tree, or checking if it’s balanced.
Graphs are another key data structure that interviewers love to explore. Questions may involve traversing graphs using DFS or BFS and finding the shortest path between two nodes using Dijkstra’s algorithm or the Bellman-Ford algorithm.
Dynamic programming is an important technique used to solve complex problems by breaking them down into smaller subproblems. Knowing how dynamic programming works can help you tackle optimization-related questions efficiently.
Understanding hash tables (or hash maps) is also crucial since they offer efficient lookup time for key-value pairs in constant time on average.
Knowing basic string manipulation techniques like reversing a string or checking if two strings are anagrams can come up during coding interviews too.
By studying these topics thoroughly and practicing solving related problems beforehand, you’ll boost your chances of acing your next Java coding interview!
D. Java Libraries and Frameworks
Java libraries and frameworks are essential tools for developers, providing pre-written code and functionality to simplify the development process. Familiarity with popular libraries and frameworks is crucial for Java coding interviews.
One commonly asked question in this category revolves around JDBC (Java Database Connectivity). Interviewers may ask about how to connect a Java application to a database using JDBC or how to handle transactions within a database.
Another important area is the Spring framework, which is widely used in enterprise-level applications. Questions could cover topics such as dependency injection, inversion of control, or the various modules within Spring like Spring MVC or Spring Boot.
Hibernate is another frequently mentioned topic in Java interviews. Questions could focus on mapping objects to relational databases using Hibernate annotations or configuring Hibernate cache strategies.
A good understanding of collections frameworks like ArrayList, HashMap, HashSet, etc., will also be tested in many interviews. You should be able to explain their differences, common use cases, and performance characteristics.
Knowledge of concurrent programming with threads and synchronization mechanisms provided by the java. util. concurrent package might come up during an interview. Understanding concepts like locks, semaphores, and atomic operations can set you apart from other candidates.
Preparing thoroughly on these library and framework topics beforehand through practice coding exercises or online courses/tutorials can greatly increase your chances of success in your next Java coding interview!
E. Debugging and Troubleshooting
Debugging and troubleshooting are crucial skills for any Java developer, as they help identify and fix errors in code. During a coding interview, you may be asked to demonstrate your ability to debug and troubleshoot Java programs. Here are some common questions that test these skills:
- How do you approach debugging a program in Java?
To debug a program, you can use tools like the Eclipse debugger or print statements to track the flow of execution and identify any issues or unexpected behavior. - What is an exception? How do you handle exceptions in Java?
An exception is an event that occurs during the execution of a program, disrupting its normal flow. In Java, exceptions can be handled using try-catch blocks to catch specific exceptions and provide appropriate error handling. - Can you explain what NullPointerException means?
A NullPointerException occurs when we try to access or call methods on objects that have not been initialized (i.e., their value is null). To avoid this error, it’s important to check if an object is null before accessing its properties or invoking methods. - Have you encountered deadlocks in your programming experience? If so, how did you resolve them?
Deadlocks occur when two or more threads get stuck waiting for each other’s resources indefinitely. To resolve deadlocks, techniques such as resource ordering and deadlock detection algorithms can be used. - What steps would you take if your application runs out of memory (OutOfMemoryError)?
If an application runs out of memory due to excessive allocation or inefficient data structures, options include optimizing code efficiency by reducing unnecessary memory usage or increasing JVM heap size through configuration settings.
Remember that demonstrating strong problem-solving skills while explaining your thought process during debugging scenarios will impress interviewers looking for candidates who can effectively troubleshoot issues when they arise.
Tips for Acing Your Java Coding Interview
Preparation is key, but it’s not just about memorizing answers. To truly ace your Java coding interview, you need to be well-rounded and confident in your skills. Here are some tips to help you succeed:
- Understand the fundamentals:
Make sure you have a solid understanding of basic Java concepts like variables, loops, conditionals, and data types. This will form the foundation of your coding knowledge. - Practice problem-solving:
Coding interviews often involve solving complex problems on the spot. Take time to practice different algorithms and data structures so that you can approach these problems with confidence. - Review object-oriented programming:
Object-oriented programming (OOP) is a fundamental concept in Java. Brush up on topics like inheritance, polymorphism, encapsulation, and abstraction to demonstrate your proficiency in OOP principles. - Familiarize yourself with common libraries and frameworks:
Many companies rely on popular libraries and frameworks in their Java projects. Be familiar with commonly used ones like Spring or Hibernate to show that you can work effectively within their ecosystem. - Debugging skills matter too:
Don’t forget about debugging! Employers want developers who can identify and fix issues efficiently. Practice debugging techniques using tools like debuggers or logging statements. - Stay calm under pressure:
Interviews can be nerve-wracking but try to stay composed during the process. Remember that it’s okay if you don’t know everything; focus on demonstrating problem-solving skills and a willingness to learn. - Communicate effectively:
During an interview, you’ll likely need to explain your thought process as you solve coding problems.
Be clear, direct, and concise when explaining your approach. Don’t hesitate to ask clarifying questions if needed.
Additional Resources for Practice and Preparation
When it comes to preparing for a Java coding interview, practice truly makes perfect. Along with studying the top 100 Java coding interview questions and answers, it’s essential to engage in hands-on practice to solidify your understanding of key concepts.
One valuable resource is online coding platforms that offer a wide range of coding challenges specifically tailored for Java. Platforms like HackerRank, LeetCode, and Codewars provide an extensive collection of Java programming problems that will test your skills and help you improve your problem-solving abilities.
Another helpful resource is participating in mock interviews or coding competitions on websites such as CodeSignal or Topcoder. These platforms not only give you exposure to real-world scenarios but also allow you to compete against other programmers, giving you a chance to gauge your performance against industry standards.
Additionally, there are numerous books available that focus specifically on preparing for Java coding interviews. Some popular titles include “Cracking the Coding Interview” by Gayle Laakmann McDowell and “Java Programming Interviews Exposed” by Noel Markham. These books provide comprehensive explanations of common interview topics along with code examples and practice exercises.
Moreover, online forums and communities dedicated to programming can be invaluable resources for obtaining guidance from experienced professionals or connecting with peers who are also preparing for their interviews. Websites like Stack Overflow or Reddit’s r/learnjava subreddit offer spaces where you can ask questions, seek advice, and learn from others’ experiences.
Don’t underestimate the power of YouTube tutorials! Many skilled instructors share their knowledge through video tutorials focused specifically on cracking coding interviews using Java. Watching these videos can enhance your understanding of complex concepts while providing practical tips and tricks for solving challenging problems.
Remember that becoming proficient in Java requires consistent effort over time. By utilizing these additional resources alongside our top 100 Java coding interview questions guide, you’ll be well-equipped to tackle any technical challenge thrown at you during your interview. Keep practicing, stay motivated, and embrace the learning process.
Conclusion:
Preparing for a Java coding interview is essential if you want to succeed and land your dream job. By familiarizing yourself with the top 100 Java coding interview questions and practicing your problem-solving skills, you can greatly increase your chances of acing the interview.
Remember to brush up on basic concepts such as variables, loops, and conditionals before diving into more complex topics like object-oriented programming, data structures, algorithms, and Java libraries. Additionally, make sure you are comfortable with debugging and troubleshooting techniques.
Utilize our comprehensive list of resources for practice and preparation to further enhance your skills. Whether it’s online tutorials, coding challenges platforms, or mock interviews – every opportunity counts in building confidence and expertise.
When it comes time for the actual interview day, remember to stay calm and composed. Take a deep breath before answering each question and take your time if needed. It’s better to provide a well-thought-out response than rushing through without fully understanding the problem.
Last but most importantly – be confident! Believe in yourself and showcase your passion for Java programming during the interview process. Employers not only look for technical knowledge but also assess how well you communicate ideas and work under pressure.
By following these tips along with thorough preparation using our curated material on Java coding interview questions, you’ll be well-equipped to excel in any Java coding interview that comes your way!
Good luck on your journey towards becoming a successful Java developer!